Develop a Simple Note Application using HTML, CSS & JavaScript โจ with some extra features ๐
Taking Notes Is An Essential Part Of Our Daily Lives, Whether It's For Work, School, Or Personal Reasons. With The Advancement In Technology, We Can Now Create Digital Notes That Are Easy To Access, Edit, And Share. In This Blog, We Will Discuss How To Create A Simple Note-Taking web app using html, CSS, JS
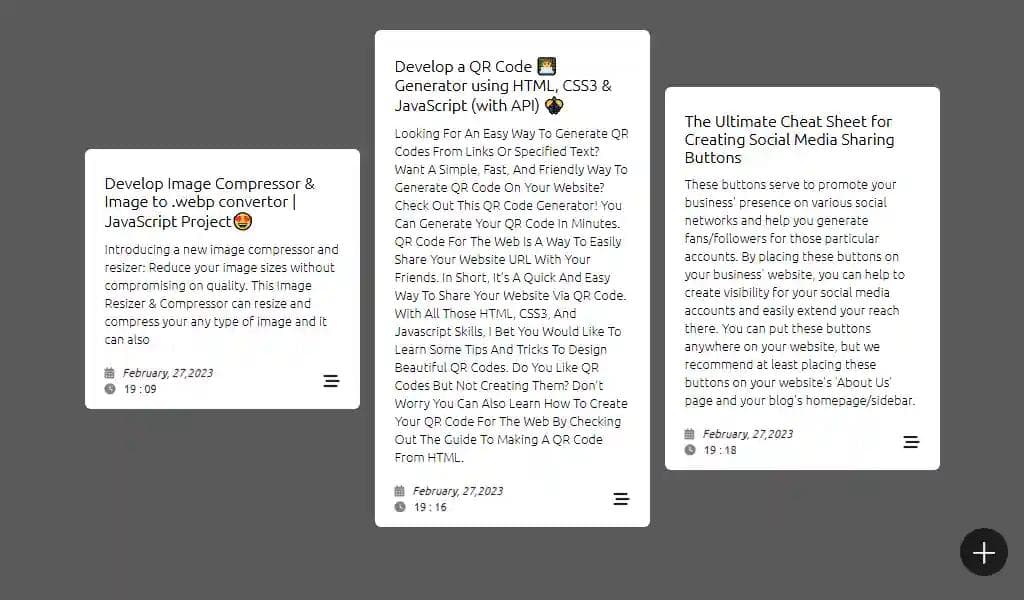
Prerequisites for this note app.
Before We Begin, Make Sure You Have A Basic Understanding Of HTML, CSS, And JavaScript. You Should Also Have A Code Editor Like Visual Studio Code Or Sublime Text Installed On Your Computer.
Features
- Create Notes: Users Will Be Able To Create New Notes By Entering The Note's Title And Description.
- Edit Notes: Users Will Be Able To Edit Their Existing Notes By Clicking On The Edit Button.
- Delete Notes: Users Will Be Able To Delete Their Notes By Clicking On The Delete Button.
- Local Storage: The Application Will Store The User's Notes On Their Browser's Local Storage So That you will not Lose your Notes Even After Closing The Browser.
- Responsive Design: The application will be mobile-friendly and responsive to different screen sizes.
Conclusion
In This Blog, You Will Learn How To Create A Simple Note-Taking Application Using HTML, CSS, And JavaScript. We Have Covered The Features Of The Application, The HTML And CSS Structure, And The JavaScript Code For Creating, Editing, & Deleting Notes And Storing Them On The User's Browser Using Local Storage. We Have Also Discussed How To Make The Application Mobile-Friendly With Responsive Design Using CSS Grid. And also You Can Customize The Application By Adding More Features And Functionalities As Per Your Needs, Like Searching Notes, Adding Tags, Categories Of Notes, etc.
Here's some code.
The HTML file you need.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>@Your-Ehsan | JS โ Note Application</title>
<link
rel="shortcut icon"
href="https://github.com/your-ehsan.png"
type="image/x-icon"
/>
<script src="https://kit.fontawesome.com/f97842efdb.js" crossorigin="anonymous"></script>
</head>
<body>
<div id="popUp_wrapper" class="popUp-wrapper">
<div class="popUp">
<div class="popUp-content">
<div class="popUp-header">
<header>
<i class="fa fa-book-bookmark"></i>
Add a new Note
</header>
<div class="closeBtn" title="Close">
<i class="fa fa-close"></i>
</div>
</div>
<form action="#">
<div class="row title">
<label for="title">Title</label>
<input
placeholder="Title for new Note"
type="text"
name="title"
id="InputTitle"
/>
</div>
<div class="row description">
<label for="description">Description</label>
<textarea
name="description"
id="InputDesc"
cols="30"
rows="10"
placeholder="Some Description For a new Note ..."
></textarea>
</div>
<button id="SaveBtn" type="button">Save Note</button>
</form>
</div>
</div>
</div>
<div class="No_Note-wrapper">
<div class="No_Note">
<figure>
<img src="./assets/images/create.png" alt="Create a Note" />
</figure>
<span>Click on <i class="fa fa-plus"></i> to add a new Note</span>
</div>
</div>
<div class="wrapper"></div>
<div title="Click To Add a new Note" id="addBox" class="addBox">
<span>Add new Note</span>
<i class="fa fa-add"></i>
</div>
</body>
</html>
All that styling you need to create this note application.
@font-face {
font-family: "ubuntu";
src: url("https://fonts.googleapis.com/css2?family=Ubuntu:wght@300&display=swap");
}
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "ubuntu";
}
body {
font-family: "ubuntu";
background: #151515b3;
filter: progid:DXImageTransform.Microsoft.gradient(startColorstr="#833ab4",endColorstr="#45fcdb",GradientType=1);
}
.addBox.hidden,
.No_Note-wrapper.hidden,
.wrapper.hidden {
display: none;
transform: scale(1);
}
.popUp-wrapper.show,
.options.show,
.addBox:hover span {
transform: scale(1);
transition: 0.3s ease-in-out;
}
.No_Note-wrapper {
width: 100%;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
text-align: center;
text-transform: capitalize;
}
.No_Note span {
color: #e7e7e7;
font-size: 1.5rem;
}
.No_Note img {
width: 200px;
}
.getOptions {
cursor: pointer;
transition: 0.3s ease-in-out;
}
.wrapper {
height: -webkit-fill-available;
padding: 30px 20px 90px 20px;
display: grid;
gap: 15px;
overflow-y: auto;
grid-template-columns: repeat(auto-fill, 275px);
align-items: center;
justify-content: center;
}
.wrapper li {
list-style: none;
background: #fff;
border-radius: 6px;
padding: 17px 20px 10px;
}
.note {
display: flex;
flex-direction: column;
justify-content: space-between;
}
.popUp-wrapper,
.addBox i {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.addBox {
position: fixed;
right: 1rem;
bottom: 0.5rem;
display: flex;
align-items: center;
cursor: pointer;
gap: 1rem;
}
.addBox i {
width: 3rem;
height: 3rem;
font: 2.5rem thin;
border-radius: 50%;
margin: 1rem 0;
background: #000000b3;
color: #fff;
}
.addBox span {
color: white;
background: #000000b3;
padding: 0.25rem 0.5rem;
border-radius: 0.2rem;
transform: scale(0);
position: relative;
font-family: "ubuntu";
}
.details {
display: flex;
justify-content: space-between;
}
.details-header {
font: 1rem bolder;
margin: 0.5rem 0;
display: -webkit-box;
-webkit-box-orient: vertical;
-webkit-line-clamp: 3;
overflow: hidden;
text-shadow: 0 0 0px black;
font-family: "ubuntu";
}
.details-text {
font: 0.8rem thin;
font-family: "ubuntu";
}
.content {
display: flex;
justify-content: space-between;
align-items: center;
color: #000000b2;
margin: 1rem 0 0;
text-shadow: 0 0 1px;
}
.content-time i {
color: #5a5a5a85;
}
.content-time span {
font: 0.7rem thin;
display: flex;
margin: 4px 0;
gap: 0.5rem;
font-family: "ubuntu";
}
.closeBtn {
cursor: pointer;
}
.getOptions {
display: flex;
justify-content: flex-end;
}
.options {
position: absolute;
background: #fff;
display: flex;
flex-direction: column;
box-shadow: -5px 5px 20px -1px black;
transform: scale(0);
transition: 0.2s ease-in-out;
margin: 2rem 0rem;
border-radius: 6px;
}
.options button {
border: none;
padding: 3px 7px;
outline: none;
text-align: right;
border-radius: 3px;
background: #fff;
cursor: pointer;
}
.options button:hover {
background: #5a5a5a3d;
}
.options button i {
margin: 3px 6px;
}
.popUp-wrapper {
position: fixed;
height: 100vh;
width: 100vw;
background: #0000004d;
transform: scale(0);
transition: 0.05s ease-in-out;
}
.popUp {
width: 320px;
background: #fff;
padding: 1rem;
border-radius: 6px;
}
.popUp-header {
display: flex;
align-items: center;
justify-content: space-between;
margin: 0 0 1rem;
}
.popUp-header i {
margin: 0.2rem 0.7rem;
font-size: 1.2rem;
}
.row {
display: flex;
flex-direction: column;
}
.title input {
padding: 0.4rem 0.5rem;
border-radius: 0.35rem;
margin: 0.5rem 0.4rem;
outline: none;
border: 1px solid;
}
.description textarea {
padding: 0.5rem 0.8rem;
margin: 0.5rem 0.4rem;
outline: none;
border: 1px solid;
border-radius: 0.3rem;
}
#SaveBtn {
border: none;
display: flex;
width: 100%;
padding: 0.7rem 0.5rem;
margin: 0.3rem 0;
justify-content: center;
border-radius: 0.3rem;
background: #000000b3;
color: white;
}
The JavaScript that is needed to add some functionalities
var D = document;
let popUp_wrapper = D.querySelector("#popUp_wrapper"),
popUp_Header = D.querySelector(".popUp-header header"),
InputTitle = D.querySelector("#InputTitle"),
InputDesc = D.querySelector("#InputDesc"),
closeBtn = D.querySelector(".closeBtn"),
SaveBtn = D.querySelector("#SaveBtn"),
addBox = D.querySelector("#addBox"),
Wrapper = D.querySelector(".wrapper");
let notes = JSON.parse(localStorage.getItem("notes") || "[]");
let months = [
"January",
"February",
"March",
"April",
"May",
"June",
"July",
"August",
"October",
"September",
"November",
"Decenmber",
];
let IsUpdated = false,
updatedID;
function Note_indicator() {
if (D.querySelector(".note")) {
D.querySelector(".No_Note-wrapper").classList.add("hidden");
Wrapper.classList.remove("hidden");
} else {
D.querySelector(".No_Note-wrapper").classList.remove("hidden");
Wrapper.classList.add("hidden");
}
}
function ShowPopUp() {
popUp_Header.innerHTML = `<i class="fa fa-book-bookmark"></i> Add a new Note`;
SaveBtn.innerHTML = "Save Note";
InputTitle.value = "";
InputTitle.focus();
InputDesc.value = "";
IsUpdated = false;
popUp_wrapper.classList.add("show");
addBox.classList.add("hidden");
}
function ClosePopUp() {
popUp_wrapper.classList.remove("show");
addBox.classList.remove("hidden");
Note_indicator();
}
addBox.addEventListener("click", ShowPopUp);
closeBtn.addEventListener("click", ClosePopUp);
function CloseOptions() {
Options.classList.remove("show");
}
function OpenOptions(e) {
e.parentElement.querySelector(".options").classList.toggle("show");
D.addEventListener("click", (i) => {
if (i.target.tagName != "I" || i.target == e) {
e.parentElement.querySelector(".options").classList.remove("show");
}
});
}
function deleteNote(noteID) {
notes.splice(noteID, 1);
localStorage.setItem("notes", JSON.stringify(notes));
AddNotes();
Note_indicator();
}
function editNote(noteID) {
ShowPopUp();
popUp_Header.innerHTML = `<i class="fa fa-edit"></i> Edit Note`;
SaveBtn.innerHTML = "Save changes";
InputTitle.focus();
InputTitle.value = notes[noteID].title;
InputDesc.value = notes[noteID].description;
IsUpdated = true;
updatedID = noteID;
}
SaveBtn.addEventListener("click", (e) => {
e.preventDefault();
let NoteTitle = InputTitle.value;
let NoteDesc = InputDesc.value;
if (NoteTitle || NoteDesc) {
let dateOBJ = new Date(),
month = months[dateOBJ.getMonth()],
day = dateOBJ.getDate(),
year = dateOBJ.getFullYear(),
Hours = dateOBJ.getHours(),
Minutes = dateOBJ.getMinutes();
if (Hours < 10) {
Hours = `0${Hours}`;
}
if (Minutes < 10) {
Minutes = `0${Minutes}`;
}
let NoteInfo = {
title: NoteTitle,
description: NoteDesc,
date: `${month}, ${day},${year}`,
time: `${Hours} : ${Minutes}`,
};
if (!IsUpdated) {
notes.push(NoteInfo);
} else {
IsUpdated = false;
notes[updatedID] = NoteInfo;
}
localStorage.setItem("notes", JSON.stringify(notes));
closeBtn.click();
AddNotes();
Note_indicator();
}
});
function AddNotes() {
D.querySelectorAll(".note").forEach((e) => e.remove());
notes.forEach((E, index) => {
let NoteHTML = `
<li class="note">
<div class="details-wrapper">
<div class="details">
<h2 class="details-header">
${E.title}
</h2>
</div>
<span class="details-text"
> ${E.description}
</span>
</div>
<div class="content">
<div class="content-time">
<span>
<i class="fa fa-calendar-alt"></i>
<em>${E.date}</em>
</span>
<span>
<i class="fa fa-clock-four"></i>
${E.time}
</span>
</div>
<div class="options-wrapper">
<div onclick="OpenOptions(this)" class="getOptions">
<i class="fa fa-bars-staggered"></i>
<div class="options">
<button onclick="editNote(${index})" class="BtnEdit" type="button">
Edit
<i class="fa fa-edit"></i>
</button>
<button onclick="deleteNote(${index})" class="BtnDelete" type="button">
Delete
<i class="fa fa-delete-left"></i>
</button>
</div>
</div>
</div>
</div>
</li>`;
Wrapper.insertAdjacentHTML("beforeend", NoteHTML);
});
}
D.addEventListener("DOMContentLoaded", () => {
AddNotes();
Note_indicator();
});